In this document
Dependencies and prerequisites
- Android 6.0 (API Level 23) or higher on the wearable and accompanying device
- Google Play services 8.3 or higher
- An Android Wear device
See also
Android 6.0 (API level 23) introduces a new permissions model, bringing some changes that are specific to Wear, and other changes that apply to all Android-powered devices.
The user must now grant permissions to Wear apps separately from the handset versions of the apps. Previously, when a user installed a Wear app, it automatically inherited the set of permissions that the user had granted to the handset version of the app. However, from Android 6.0 (API level 23), the Wear app no longer inherits these permissions. Thus, for example, a user might grant a handset app permission to use location data, and subsequently have to grant the same permission to the Wear version of the app.
For both Wear and handset apps, the Android 6.0 (API level 23) permissions model also streamlines app installation and upgrade by eliminating the requirement that the user grant upfront every permission an app may ever need. Instead, the app does not request permissions until it actually needs them.
Note: For an app to use the new permissions model, it must
specify a value of 23
for both
uses-sdk-element
and compileSdkVersion
.
The rest of this document discusses how to use the Android 6.0 (API level 23) permissions model when developing Android Wear apps.
Permission Scenarios
Broadly speaking, there are four scenarios you may encounter when requesting dangerous permissions on Android Wear:
- The Wear app requests permissions for an app running on the wearable device.
- The Wear app requests permissions for an app running on the handset.
- The handset app requests permissions for an app running on the wearable device.
- The wearable app uses a different permission model from its handset counterpart.
The rest of this section explains each of these scenarios. For more detailed information about requesting permissions, see Requesting Permissions.
Wear app requests permission for an app running on the wearable device
When the Wear app requests a permission for an app running on the wearable device, the system
displays a dialog to prompt the user for that permission. An app or service can only call the
requestPermissions()
method from an activity. If the user interacts with your app
via a service, such as
a watch face, the service must open an activity before requesting the permission.
Your app requests permissions in context when it’s clear why the permissions are needed to perform a given operation. If it's obvious that your app requires certain permissions, your app can prompt for them on launch. If it may not be so obvious, you may choose to provide additional education before prompting for a permission.
If an app or watch face requires more than one permission at a time, permission requests appear one after the other.
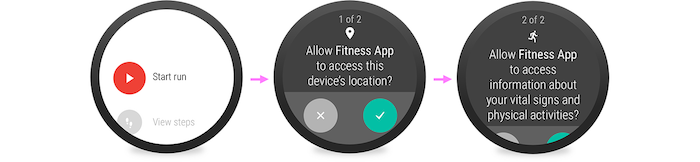
Figure 1. Permission screens appearing in succession.
Note: From Android 6.0 (API level 23), Android Wear automatically syncs Calendar, Contact, and Location data to the Wear device. As a result, this scenario is the applicable one when Wear requests this data.
Wear app requests handset permission
When the Wear app requests a handset permission, the Wear app must send the user to the handset to accept the permission. There, the handset app can provide additional education to the user via an activity. The activity should include two buttons: one for granting, and one for denying, the permission.
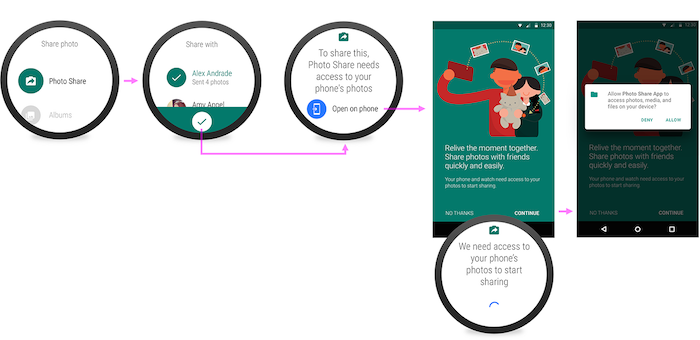
Figure 2. Sending the user to the handset to grant permission.
Handset app requests wearable permission
When the user is in a handset app and the app requires a wearable permission, the
handset app must send the user to the wearable to accept the permission.
The handset app uses the
requestPermissions()
method on the wearable to trigger the system permissions dialog.
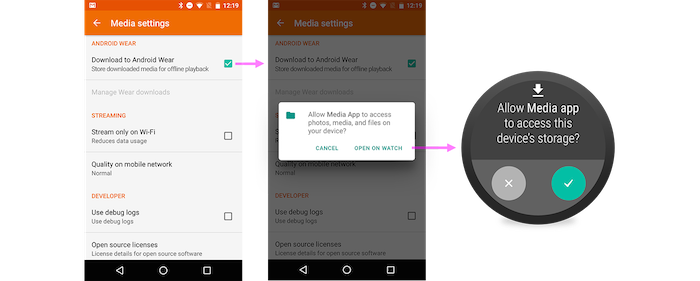
Figure 3. Sending the user to the wearable to grant permission.
Mismatching permission models between wearable and handset app
If your handset app begins using the Android 6.0 (API level 23) model but your wearable app does not, the system downloads the Wear app, but does not install it. The first time the user launches the app, the system prompts them to grant all pending permissions. Once they do so, it installs the app. If your app, for example a watch face, does not have a launcher, the system displays a stream notification asking the user to grant the permissions the app needs.
Permission-Request Patterns
There are different patterns for requesting permission from users. In order of priority, they are:
- Ask in context when the permission is obviously necessary for a specific functionality, but is not necessary for the app to run at all.
- Educate in context when the reason for requesting the permission is not obvious, and the permission is not necessary for the app to run at all.
- Ask up front when the need for the permission is obvious, and the permission is required in order for the app to run at all.
- Educate up front when the need for the permission is not obvious, but the permission is required in order for the app to run at all.
Ask in context
Your app should request permissions when it’s clear why they are needed in order to perform a given operation. Users are more likely to grant a permission when they understand its connection to the feature they want to use.
For example, an app may require a user’s location in order to show nearby places of interest. When the user taps to search for nearby places, the app can immediately request the location permission, because there is a clear relationship between searching for nearby places and the need for the location permission. The obviousness of this relationship makes it unnecessary for the app to display additional education screens.
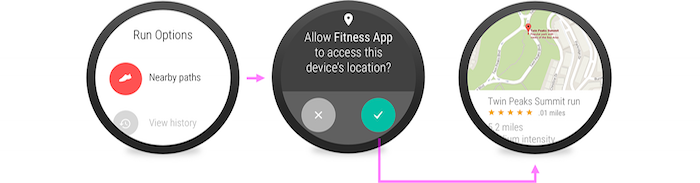
Figure 4. Asking in context.
Educate in context
If necessary, you may choose to provide additional education before prompting for a permission. Again, your app should do this in context of a specific action, if it’s unclear why your app needs access to the requested permission in order to complete that action.
Figure 5 shows an example of in-context education. The app does not require permissions in order to start the timer, but an inline educational cue shows that part of the activity (location detection) is locked. When the user taps the cue, a permission-request screen appears, allowing the user to unlock location-detection.
You can use the shouldShowRequestPermissionRationale()
method to help your app decide whether to provide more
information. For additional details, see Requesting Permissions
at Run Time.
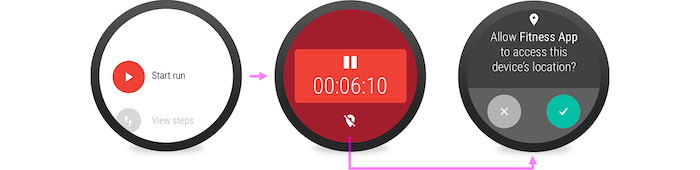
Figure 5. Educating in context.
Ask up front
If your app clearly requires a permission in order to work at all, you can prompt for that permission when the user launches the app. For example, a maps app clearly requires access to the device’s location to run its expected activities. No further education is necessary for this permission.
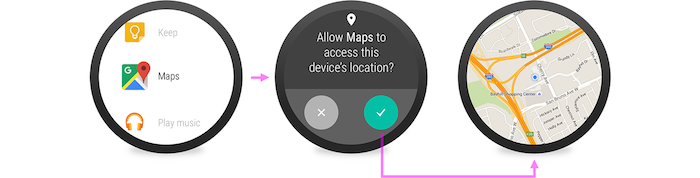
Figure 6. Asking up front.
Educate up front
In some cases, the app requires a permission for basic functionality, but the need for that permission is not obvious. In these cases, when the user first starts the app or sets a watch face, the app or watch face may choose to educate the user and ask for the permission.
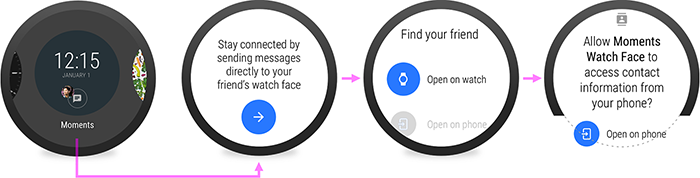
Figure 7. Educating up front.
Handling Rejection
If a user denies a requested permission that is not critical to an intended activity, do not block them from continuing the activity. If certain parts of the activity are disabled by the denied permission, provide visual, actionable feedback. Figure 8 shows the use of a lock icon to indicate that a feature is locked because the user did not grant permission to use it.
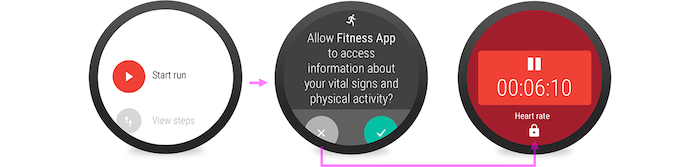
Figure 8. Lock icon, showing a feature is locked because of denied permission.
When a previously denied wearable permission dialog appears a second time, it includes a Deny, don't show again option. If the user chooses this option, then the only way for them to allow this permission in the future is to go into the wearable's Settings app.
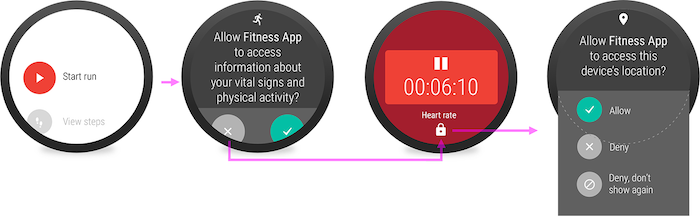
Figure 9. Offering not to show the permission-request screen anymore.
Permissions for Services
As mentioned above, only an activity can call the
requestPermissions()
method, so if the user interacts with your app via a service,
for example a watch face, the service must open a background activity before requesting
the permission. This activity could provide additional education, or it could simply
be an invisible activity that brings up the system dialog.
If your wearable app runs a service that is not a watch face, and the user does not launch an app in which it might make sense to request a permission, you can post an educational notification on the wearable. The notification can provide an action to open an activity that then triggers the system permissions dialog.
Note: This is the only acceptable use of a stream notification for permissions requests.
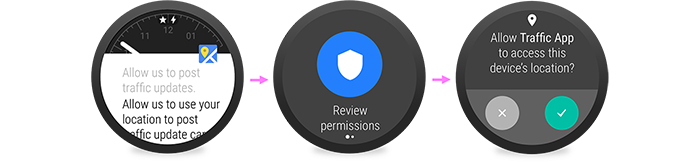
Figure 10. A service requesting permission.
Settings
As with the handset, the user can change a Wear app’s permissions in Settings at any time.
Therefore, when the user tries to do something that requires a
permission, the app should always first call the
checkSelfPermission()
method to see if the app currently has permission to perform this operation. The app should perform
this check even if it knows the user has previously granted that permission, since the
user might have subsequently revoked that permission.
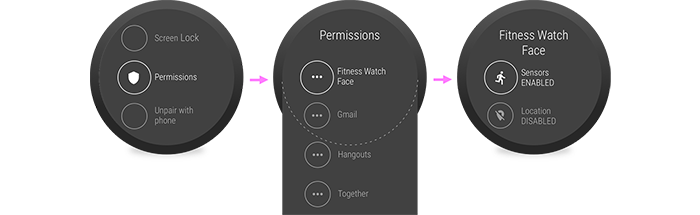
Figure 11. Changing settings via the Settings app.